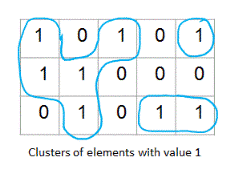
https://leetcode.com/problems/number-of-islands/solution/
Problem Statement:
Given a 2d grid map of ‘1’s (land) and ‘0’s (water), count the number of islands. An island is surrounded by water and is formed by connecting adjacent lands horizontally or vertically. You may assume all four edges of the grid are all surrounded by water.
Examples
1:
Input: grid = [
["1","1","1","1","0"],
["1","1","0","1","0"],
["1","1","0","0","0"],
["0","0","0","0","0"]
]
Output: 1
2:
Input: grid = [
["1","1","0","0","0"],
["1","1","0","0","0"],
["0","0","1","0","0"],
["0","0","0","1","1"]
]
Output: 3
Idea:
This question is analogous to the problem of connected components in an undirected graph.
Here I used recursion for a depth first search.
Solution:
DFS:
void dfs(int i, int j, vector<vector<char>>& grid) {
// exit if search exhuasted
if(i < 0 || j < 0 || i >= grid.size() || j >= grid[0].size() || grid[i][j] != '1')
return;
grid[i][j] = '0';
dfs(i - 1, j, grid);
dfs(i, j - 1, grid);
dfs(i + 1, j, grid);
dfs(i, j + 1, grid);
}
int numIslands(vector<vector<char>>& grid) {
for(unsigned i = 0; i < grid.size(); ++i) {
for(unsigned j = 0; j < grid[0].size(); ++j) {
if(grid[i][j] == '1') {
dfs(i, j, grid);
++count;
}
}
}
return count;
}
Union Find:
int numIslands(vector<vector<char>>& grid) {
}
Complexity Analysis:
Time
Worst case is O(M X N) where M and N are the dimensions of the input grid.
Memory
Here we reuse the input grid which is good. However since it is recursive, its possible to use O(MxN) memory in the case where the grid is all ones.
Comments
Nothing yet.